MicroPython 'os' Module
Contents
Introduction
The micro:bit's MicroPython documentation is generally quite good. However it doesn't cover the 'pure' language in any detail - a gap that this website attempts to remedy.
Additionally there are MicroPython modules available to the micro:bit that aren't documented. In the case of the os module it is documented but not all of the available functions are described - thus the reason for this webpage.
This tutorial will take most of its material from the official MicroPython for micro:bit documentation, but fill in the gaps and provide working examples that have been run on a micro:bit.
The os Module's Purpose
MicroPython contains an os module based upon the os module in the Python standard library. It's used for accessing what would traditionally be termed as operating system dependent functionality. Since there is no operating system on the micro:bit the module provides functions relating to the management of the simple on-device persistent file system and information about the current system.
As is the case for using all modules in MicroPython an import ststement is needed:
import os
Since the os module allows access to the micro:bit's very basic MicroPython file system, for program test purposes it is necessary to firstly ensure that there are several files available on the device - three or four would be ideal.
This tutorial will use the Mu Editor to test and run all code examples. The Mu Editor has a Files button on the toolbar that allows transfer of files between the micro:bit and the attached computer in both directions.
Connect the micro:bit to the computer and start the Mu Editor. Click the Files button to display the micro:bit's MicroPython filesystem. There should be a file main.py which is the last MicroPython program to have run on the device. Ensure that the micro:bit (left pane) has three or more files listed. More can be copied over, if necessary, by dragging files from the right pane (computer) to the left pane (micro:bit).
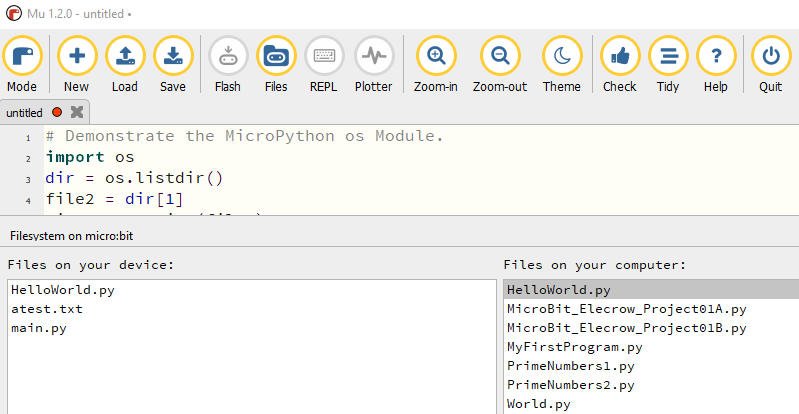
The os Module's Functions
The os module for the micro:bit exposes six functions:
os.listdir()
Returns a list of the names of all the files contained within the local persistent on-device file system.
Example:
# Demonstrate os.listdir() function
import os
dir = os.listdir()
print('Directory listing:')
print(dir)
print('Number of files:', len(dir))
Output: ['HelloWorld.py', 'atest.txt', 'main.py'] Number of files: 3
os.ilistdir()
Returns an iterator with details of the files contained within the local persistent on-device file system.
Each element returned by the iterator is a tuple with three values:
- File name
- File (32768) or directory (16384); The micro:bit file system is flat with no subdirectories so this value will always be 32768.
- inode; this is a Linux concept that the micro:bit doesn't understand so will always be 0
Example:
# Demonstrate os.ilistdir() function
import os
dir = os.ilistdir()
count = 0
print('Directory listing:')
for file in dir:
print(file)
count += 1
print('Number of files:', count)
Output: Directory listing: ('main.py', 32768, 0) ('HelloWorld.py', 32768, 0) ('atest.txt', 32768, 0) Number of files: 3
os.size(filename)
Returns the size, in bytes, of the file named in the argument filename. If the file does not exist an OSError exception will occur.
Example:
# Demonstrate os.size() function
# Report all file names and file size
import os
dir = os.ilistdir()
print('Directory listing:')
for file in dir:
name = file[0]
print(name, end=' ')
print(os.size(name), 'bytes')
print()
# An exception occurs if file name
# is not found.
badsize = os.size('foo')
Output: Directory listing: main.py 249 bytes HelloWorld.py 294 bytes atest.txt 25 bytes Traceback (most recent call last): File "main.py", line 14, in <module> OSError: [Errno 2] ENOENT
os.stat(filename)
Returns a tuple of 10 elements with information on the given file.
The first element of the tuple ([0]) will always return 32768 indicating the filesystem item is a file. The simple micro:bit filesystem does not allow directories.
The seventh element of the tuple ([6]) is the file size in bytes.
All other elements of the tuple have a value of 0 as the underlying attribute has no meaning on the micro:bit.
Example:
# Demonstrate os.stat() function
import os
Stat = os.stat('main.py')
print(Stat)
print('file size:', Stat[6])
Output: (32768, 0, 0, 0, 0, 0, 113, 0, 0, 0) file size: 113
os.remove(filename)
Removes (deletes) the file named in the argument filename. If the file does not exist an OSError exception will occur.
Example:
# Demonstrate os.remove() function
import os
# add a new text file to the
# micro:bit's filesystem
filename = 'spam.txt'
f = open(filename, 'wt')
f.write('Hello World')
f.close
# Show the new file in
# a directory listing.
count = 0
dir = os.ilistdir()
print('Directory listing:')
for file in dir:
print(file[0])
count += 1
print('Total files:', count)
# Delete the new file
os.remove(filename)
# Print another directory listing
# to show that the new file
# is deleted.
count = 0
dir = os.ilistdir()
print('\nDirectory listing:')
for file in dir:
print(file[0])
count += 1
print('Total files:', count)
# Attempting to delete a file
# that doesn't exist will throw
# an exception (error).
print()
os.remove('bad_file')
Output: Directory listing: main.py spam.txt HelloWorld.py atest.txt Total files: 4 Directory listing: main.py HelloWorld.py atest.txt Total files: 3 Traceback (most recent call last): File "main.py", line 39, in <module> OSError: [Errno 2] ENOENT
os.uname()
Returns information identifying the current operating system. The return value is a tuple with five attributes:
- sysname - operating system name
- nodename - name of machine on network (implementation-defined)
- release - operating system release
- version - operating system version
- machine - hardware identifier
Example:
# Demonstrate os.uname() function
import os
sys = os.uname()
print('sysname:', sys[0])
print('nodename:', sys[1])
print('release:', sys[2])
print('version:', sys[3])
print('machine:', sys[4])
Output: sysname: microbit nodename: microbit release: 2.1.2 version: micro:bit v2.1.2+0697c6d on 2023-10-30; MicroPython v1.18 on 2023-10-30 machine: micro:bit with nRF52833